Your cart is currently empty!
Python and JavaScript are not the same, and yet, I keep seeing Python code that reeks of JavaScript habits. Stop it. Just stop. If you write Python like it’s JavaScript, you’re making life harder for everyone—including yourself.
JavaScript is designed for asynchronous, event-driven environments, while Python prioritizes readability, simplicity, and explicitness. The patterns that work well in one don’t always translate to the other. Let’s call out these bad habits, explain why they’re a problem, and fix them with proper Pythonic alternatives.
Table of Contents
- 1. Stop Using camelCase Like You're Writing React Components
- 2. Not Everything Needs to Be a Damn Function Factory
- 3. Python Isn't Just a Collection of Dictionaries
- 4. Chill With the lambda Overuse
- 5. Async Code in Python Isn’t JavaScript’s async/await
- 6. List Comprehensions Are Not a Personality Trait
- 7. print() Isn’t Your Debugging Tool, Use logging
- JavaScript Habits Don’t Belong in Python
1. Stop Using camelCase Like You’re Writing React Components
In JavaScript, camelCase is the norm because of its deep integration with objects and JSON. But Python has its own idiomatic standard: snake_case. This isn’t just an arbitrary rule—it improves readability, consistency, and integration with Python’s standard library, which also follows snake_case.
Bad (JavaScript-style naming in Python):
userProfile = {"name": "Grogu", "age": 50}
def getUserData():
return userProfile["name"]
Good (Pythonic naming):
user_profile = {"name": "Grogu", "age": 50}
def get_user_data():
return user_profile["name"]
Consistent naming conventions improve readability and prevent cognitive overload when jumping between different projects.
2. Not Everything Needs to Be a Damn Function Factory
JavaScript relies on function factories and closures to encapsulate scope, especially since var
has function-level scope instead of block scope. But in Python, you don’t need to overcomplicate simple operations with nested function factories.
Bad (JavaScript-style function factory):
def make_adder(x):
def adder(y):
return x + y
return adder
add_five = make_adder(5)
print(add_five(10)) # 15
This is unnecessary in most Python use cases. Instead, just use a plain function:
Good (Pythonic approach):
def add(x, y):
return x + y
print(add(5, 10)) # 15
Unless you’re working with higher-order functions or decorators, closures are overkill in Python.
3. Python Isn’t Just a Collection of Dictionaries
JavaScript developers often default to object literals ({}
) to store structured data because JSON is a native format. But Python has more robust tools like dataclasses, which provide type safety and better maintainability.
Bad (obsession with dictionaries):
settings = {
"theme": "dark",
"notifications": True,
"volume": 80
}
print(settings["theme"])
Dictionaries are fine for dynamic configurations, but for structured data, use a dataclass:
Good (use dataclass
instead):
from dataclasses import dataclass
@dataclass
class Settings:
theme: str
notifications: bool
volume: int
settings = Settings("dark", True, 80)
print(settings.theme)
A dataclass
provides better organization, type hinting, and validation.
4. Chill With the lambda
Overuse
JavaScript developers overuse arrow functions because they make callbacks and event-driven code more concise. But Python doesn’t require the same level of terseness.
Bad (unnecessary lambda):
add = lambda x, y: x + y
print(add(3, 5))
Lambdas should only be used for inline, throwaway functions. If the function is more than one operation, just define it properly:
Good (just use def
):
def add(x, y):
return x + y
print(add(3, 5))
Explicitly named functions make debugging and code comprehension easier.
5. Async Code in Python Isn’t JavaScript’s async/await
JavaScript’s async/await
is deeply tied to its single-threaded event loop. In Python, async programming requires asyncio
, which must be handled correctly.
Bad (JavaScript-style async handling):
async def fetch_data():
return "data"
def main():
data = fetch_data()
print(data)
main()
This won’t work because fetch_data()
returns a coroutine, not the actual result. You need to await it properly:
Good (Pythonic async handling):
import asyncio
async def fetch_data():
return "data"
async def main():
data = await fetch_data()
print(data)
asyncio.run(main())
Python’s async model is different—learn the nuances.
6. List Comprehensions Are Not a Personality Trait
List comprehensions are great, but overusing them for complex operations hurts readability.
Bad (overcomplicated one-liner):
numbers = [x * x for x in range(10) if x % 2 == 0]
Good (write readable code):
def square_evens(numbers):
results = []
for num in numbers:
if num % 2 == 0:
results.append(num * num)
return results
print(square_evens(range(10)))
Readability matters more than cleverness.
7. print()
Isn’t Your Debugging Tool, Use logging
JavaScript developers rely on console.log()
for debugging, but Python has a built-in logging module that scales much better.
Bad (JavaScript-style debugging):
print("Debug info:", data)
Good (Pythonic logging):
import logging
logging.basicConfig(level=logging.DEBUG)
logging.debug("Debug info: %s", data)
Logging allows you to control output levels, write logs to files, and debug efficiently.
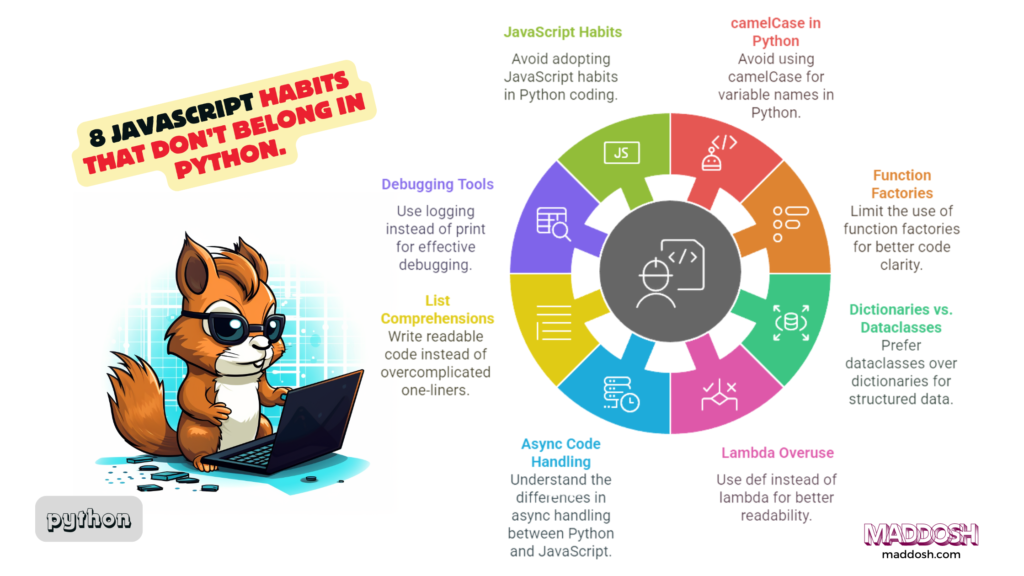
JavaScript Habits Don’t Belong in Python
Python is not JavaScript. Each language has its own ecosystem, strengths, and conventions. Writing Python like JavaScript makes your code harder to read, harder to maintain, and just plain annoying to work with.
Write Python like Python. Use snake_case
, embrace Pythonic structures, and understand Python’s async model before diving in headfirst. If you’re making the transition from JavaScript to Python, respect the differences.
Got more JavaScript-inspired Python sins? Drop them in the comments—let’s fix them together.
Staff picks
-
$21.00
Squirrel Sunglasses Tee
-
$21.00
Crayon Raccoon Tee
-
$21.00
Koala Sunglasses Tee
-
$21.00
Bear Graphic Tee
-
$21.00
Wolf Tee – Colorful Crayon Drawing, Unisex Heavy Cotton Shirt
-
$21.00
Deer Sunglasses Tee
Debugging Circular Imports in Python: Clean Project Layout
Circular imports are one of the most common, annoying, and avoidable problems…
Debugging Python AsyncIO Errors: Event Loop Problems Solved
AsyncIO is deceptively simple—until it isn’t. You’re probably here because you hit…
How to Fix Python Memory Leaks With tracemalloc
Struggling with a Python app that keeps eating up memory? Learn how…
Fixing “ModuleNotFoundError” in Python (Fast Debugging Guide)
Struggling with Python’s dreaded ModuleNotFoundError? This fast debugging guide covers exactly why…
How to Resolve ImportErrors and ModuleNotFoundErrors in Python Projects
Struggling with Python import errors? Learn how to fix ImportError and ModuleNotFoundError…
Stop Writing Python Like JavaScript – Common Mistakes and How to Fix Them
Python and JavaScript are not the same, and yet, I keep seeing…
Leave a Reply