Your cart is currently empty!
Python is loved for its simplicity and readability, but let’s be real—it’s not the fastest language out there. If you’re working with large datasets, backend APIs, or performance-critical applications, you need serious optimizations to avoid slow execution times, high memory usage, and bottlenecks.
This guide will cover Python performance optimization tips that actually work—from profiling bottlenecks to leveraging built-in functions and memory-efficient techniques.
Ready to make Python run faster? Let’s go. 🚀
Table of Contents
- What Does It Mean to Optimize Python?
- How Python Works Under the Hood (And Why It’s Slower Than C or Java)
- Where Does Python Optimization Matter Most?
- When Should You Optimize Python? (And When You Shouldn't)
- 1. Profile Before You Optimize (Stop Guessing, Start Measuring)
- 2. Use Built-in Functions Instead of Manual Loops
- 3. Avoid Using for Loops on Large Data (Use NumPy Instead)
- Reduce Memory Usage with Generators Instead of Lists
- 5. Use multiprocessing to Utilize All CPU Cores
- 6. Cache Function Results to Avoid Recomputing (lru_cache)
- 7. Optimize String Operations with join() Instead of + in Loops
- Optimize or Be Left Behind
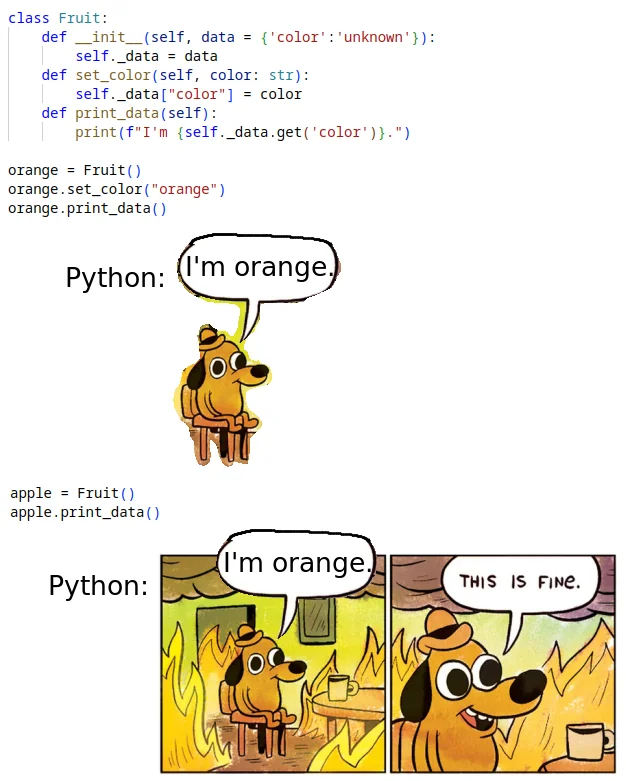
Why Do We Need to Optimize Python?
Python is elegant and readable, but it’s not always fast. Unlike lower-level languages like C, Rust, or even Java, Python prioritizes developer productivity over raw execution speed. This tradeoff makes Python easy to write, but it can become painfully slow when handling large datasets, high-throughput applications, or complex computations.
If you’ve ever had a Python script run fine on small inputs but slow to a crawl on real-world data, you’ve already experienced this firsthand. Optimization isn’t just about making Python faster—it’s about making it efficient for real-world use cases.
What Does It Mean to Optimize Python?
Optimization in Python means reducing execution time, memory usage, or computational overhead while maintaining the same functionality. You can optimize Python code in several ways, including:
🔹 Algorithmic Optimization – Choosing better data structures and efficient algorithms instead of brute-force approaches.
🔹 Code-Level Optimization – Using Pythonic features like list comprehensions, built-in functions, and avoiding unnecessary loops.
🔹 Memory Optimization – Reducing RAM usage with generators, NumPy arrays, or structuring data more efficiently.
🔹 Parallelization & Concurrency – Using multiprocessing and threading to take advantage of multi-core CPUs.
🔹 Profiling & Debugging – Finding real bottlenecks instead of blindly tweaking code.
In short, optimization is about writing smarter, not just faster.
How Python Works Under the Hood (And Why It’s Slower Than C or Java)
Python’s flexibility comes from being interpreted, dynamically typed, and memory-managed, but these features also introduce performance tradeoffs:
🔹 Global Interpreter Lock (GIL) – Python only executes one thread at a time, making multi-threading ineffective for CPU-bound tasks.
🔹 Dynamic Typing Overhead – Unlike C/C++, Python has to constantly check variable types at runtime, adding processing overhead.
🔹 High-Level Abstraction – Python’s built-in functions are convenient, but they come with extra processing layers compared to low-level languages.
Despite these drawbacks, Python can still be optimized significantly with the right techniques.
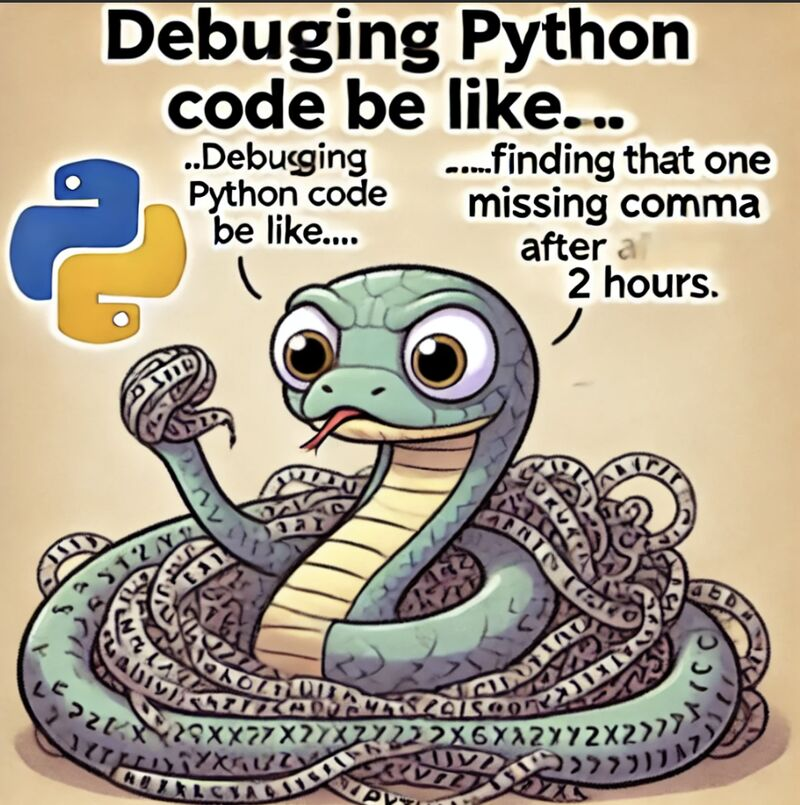
Where Does Python Optimization Matter Most?
Optimization isn’t always necessary, but in some fields, it’s a dealbreaker.
✅ Data Science & Machine Learning – Training and analyzing large datasets needs fast execution. Python is often paired with NumPy, Pandas, and C-accelerated libraries to avoid slow loops.
✅ Backend APIs & Web Development – APIs should respond in milliseconds, not seconds. Optimizing query handling, caching, and response times is crucial for fast web apps.
✅ Automation & Scripting – If you’re automating workflows, efficiency matters. Slow scripts waste developer time and server resources.
✅ Financial & Scientific Computing – Finance, physics simulations, and AI modeling often require high-performance numerical processing.
✅ Game Development & Graphics Processing – Although Python isn’t ideal for game engines, optimization helps in game scripting, AI, and data handling.
📌 If your Python code processes massive data, runs in real-time, or interacts with users, optimization matters.
When Should You Optimize Python? (And When You Shouldn’t)
Optimize only when necessary. Not every script needs extreme performance tuning. Sometimes, cleaning up messy code gives more benefit than premature optimization.
🚨 When to Optimize:
✔ Your script is too slow on large inputs (e.g., ML training, data parsing).
✔ Your API response times are lagging and impacting user experience.
✔ Your script is using excessive memory, leading to crashes.
✔ You need high-performance processing, like video analysis or real-time analytics.
⛔ When NOT to Optimize:
❌ Your code runs fast enough for its use case.
❌ You sacrifice readability for micro-speed improvements.
❌ You haven’t profiled your code yet (guessing ≠ optimizing).
🛑 Rule of Thumb: Write clean code first, optimize when necessary.
Alright. Enough chatting. Let’s get into our top 5 ways to optimize your Python code for maximum performance.
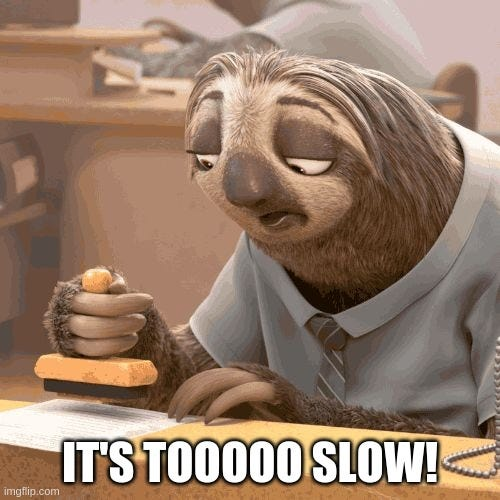
1. Profile Before You Optimize (Stop Guessing, Start Measuring)
Why You Should Care.
Most developers waste time optimizing the wrong part of their code. If you don’t measure what’s actually slow, you’ll end up blindly rewriting functions that aren’t the real bottleneck.
Imagine spending hours rewriting a sorting algorithm, only to realize that the real issue was an inefficient database query.
Python provides profiling tools that show exactly which functions are slowing you down. By measuring first, you can focus your efforts on real bottlenecks instead of wasting time on micro-optimizations that won’t make a difference.
Bad Code (Guessing Instead of Profiling)
pythonCopyEditfor i in range(10_000_000): # Why is this slow? Who knows...
do_something(i)
How to Fix It (Use cProfile
to Find Slow Functions)
pythonCopyEditimport cProfile
def slow_function():
result = sum(range(10_000_000)) # Example slow operation
cProfile.run('slow_function()')
🔍 What This Does:
- Runs your function while tracking execution time.
- Shows which parts of your code are actually slow.
- Helps you prioritize the biggest bottlenecks first.
💡 Pro Tip: Make Profiling Visual
For huge codebases, use SnakeViz to get interactive graphs:
bashCopyEditpip install snakeviz
python -m cProfile -o output.prof my_script.py
snakeviz output.prof
This will show you visually where time is wasted. Fixing one big bottleneck can instantly speed up everything.
2. Use Built-in Functions Instead of Manual Loops
Why You Should Care
Python’s built-in functions are written in C and optimized for performance. Manually looping through lists when a built-in function can do the job is wasting CPU cycles.
This ties back to writing Pythonic code. If you’re still manually iterating over lists instead of using list comprehensions, map()
, or zip()
, you’re slowing yourself down for no reason. A cleaner, more efficient codebase is naturally faster.
Bad Code (Manual Looping = Slower Execution)
pythonCopyEditnumbers = [1, 2, 3, 4, 5]
squared = []
for num in numbers:
squared.append(num ** 2)
Better Code (Use List Comprehensions)
pythonCopyEditsquared = [num ** 2 for num in numbers]
✅ Why This Is Faster: List comprehensions execute at C speed, making them faster than manually appending in loops.
Even Faster? Use map()
pythonCopyEditsquared = list(map(lambda x: x ** 2, numbers))
🔹 When to Use map()
? If you’re applying a function to a dataset and need raw speed.
3. Avoid Using for
Loops on Large Data (Use NumPy Instead)
Why You Should Care
If you’re dealing with huge amounts of numerical data, plain Python lists are painfully slow. Instead of manually iterating over numbers, NumPy handles operations in highly optimized C code, making it 50-100x faster.
Imagine processing millions of financial transactions or analyzing large datasets. If you stick with standard lists, your script will take minutes instead of seconds.
Bad Code (Using Python Lists for Computation = Slow)
pythonCopyEditnumbers = list(range(1_000_000))
squared = [x ** 2 for x in numbers]
Better Code (Use NumPy for Vectorized Computation)
pythonCopyEditimport numpy as np
numbers = np.arange(1_000_000)
squared = numbers ** 2
📌 Performance Boost:
✅ Regular Python list: ~800ms
✅ NumPy array: ~10ms (80x faster!)4. Reduce Memory Usage with Generators Instead of Lists
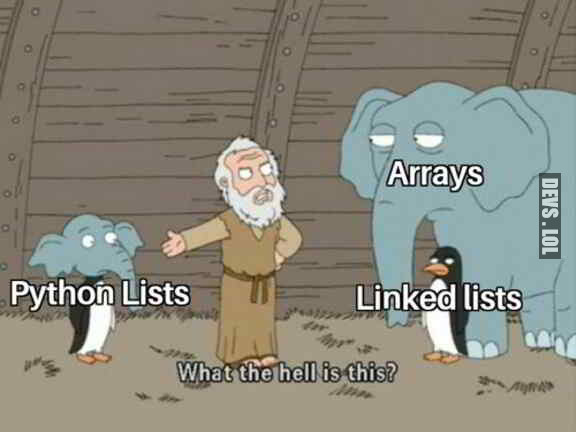
Reduce Memory Usage with Generators Instead of Lists
Why You Should Care
If you’re processing millions of records or working with large datasets, storing everything in a list can eat up memory fast. This can lead to:
- High RAM usage 🛑
- Slow execution times ⏳
- Potential crashes 💥
Instead of loading everything at once, generators let you yield values one at a time, keeping memory usage low.
Bad Code (Using a List for Large Data Processing)
pythonCopyEditdata = [process(x) for x in range(10_000_000)] # This consumes a lot of RAM
Better Code (Use a Generator Instead)
pythonCopyEditdef process_data():
for x in range(10_000_000):
yield process(x)
data = process_data() # Uses almost NO memory!
✅ Why This Is Faster:
- Generates values on the fly instead of storing them in memory.
- Ideal for large-scale data processing, log handling, and streaming APIs.
📌 When to Use Generators?
✔ If you’re processing huge datasets (e.g., reading logs, analyzing CSVs).
✔ When your script only needs one item at a time.
✔ For infinite sequences (e.g., generating unique IDs dynamically).
⚠ When NOT to Use Generators?
❌ If you need to access the entire dataset at once.
❌ When you need random access (e.g., sorting a dataset before processing).
🚀 Performance Boost: Generators use almost zero memory compared to lists, making them a must-have for scalable Python applications.
5. Use multiprocessing
to Utilize All CPU Cores
Why You Should Care
Python only runs one thread at a time due to the Global Interpreter Lock (GIL). If your script is CPU-heavy (e.g., data crunching, image processing), it will only use one core, leaving the rest of your CPU wasting power.
Using the multiprocessing
module, you can distribute tasks across multiple CPU cores, massively speeding up execution.
Bad Code (Single-Threaded Execution, Wasting CPU Power)
pythonCopyEditdef compute(x):
return x ** 2
results = [compute(x) for x in range(1_000_000)]
Better Code (Use multiprocessing
for Faster Execution)
pythonCopyEditfrom multiprocessing import Pool
def compute(x):
return x ** 2
with Pool() as pool:
results = pool.map(compute, range(1_000_000))
✅ Why This Is Faster:
- Runs on multiple CPU cores instead of just one.
- Massively speeds up computations for large datasets.
📌 When to Use multiprocessing
?
✔ If your task is CPU-bound (e.g., heavy computations, image processing).
✔ When working with large data that can be processed in chunks.
⚠ When NOT to Use multiprocessing
?
❌ If your task is I/O-bound (e.g., waiting for network responses). Use asyncio
instead.
❌ If processes share complex objects—multiprocessing
doesn’t handle shared state well.
📌 Performance Benchmark:
✅ Single-threaded: 5 seconds
✅ Multiprocessing (4 cores): ~1 second (5x faster!)
🚀 If you’re not using multiprocessing
for CPU-heavy tasks, you’re leaving performance on the table.
6. Cache Function Results to Avoid Recomputing (lru_cache
)
Why You Should Care
If your function recalculates the same values multiple times, you’re wasting CPU cycles. Instead of recomputing expensive function calls, cache the results and return them instantly when needed.
Imagine you have a function that fetches data from an API or performs a slow recursive calculation—instead of running it from scratch each time, caching saves previous results for faster access.
Bad Code (Repeated Expensive Computations)
pythonCopyEditdef slow_function(x):
print("Computing...")
return x ** 2
slow_function(10) # Runs the computation
slow_function(10) # Runs again (wasted time)
Better Code (Use lru_cache
to Store Previous Results)
pythonCopyEditfrom functools import lru_cache
@lru_cache(maxsize=100)
def slow_function(x):
print("Computing...")
return x ** 2
slow_function(10) # First time: Computes
slow_function(10) # Second time: Instantly returns cached result
✅ Why This Is Faster:
- The first call computes the result normally.
- The second call retrieves the result from the cache instead of recomputing.
- Works great for recursive functions, API calls, and CPU-heavy calculations.
📌 When to Use Caching?
✔ If a function is called multiple times with the same inputs.
✔ When dealing with slow computations (e.g., Fibonacci, web scraping, database queries).
✔ If data doesn’t change frequently (cache invalidation is important!).
⚠ When NOT to Use Caching?
❌ If your function depends on real-time data (e.g., live stock prices).
❌ If your function returns huge data structures—caching them takes up memory.
🚀 Performance Boost: Cached functions can run 100x faster, reducing redundant computation significantly!
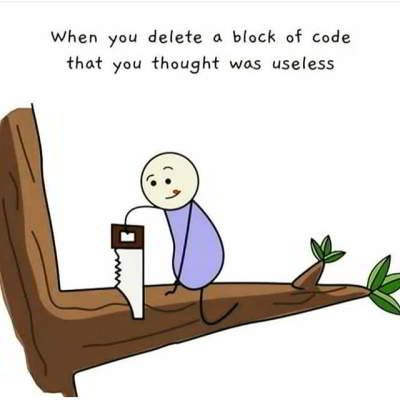
7. Optimize String Operations with join()
Instead of +
in Loops
Why You Should Care
Python strings are immutable, meaning every time you use +
to concatenate strings, a new string is created in memory. This can slow down large loops significantly.
If your code involves building long text files, processing logs, or creating large reports, inefficient string handling will crush performance.
Bad Code (String Concatenation in a Loop = Slow Memory Allocation)
pythonCopyEditsentence = ""
words = ["Python", "is", "awesome"]
for word in words:
sentence += word + " " # Creates a new string object each iteration (slow!)
Better Code (Use .join()
for Efficient Concatenation)
pythonCopyEditsentence = " ".join(words)
✅ Why This Is Faster:
- Concatenates in one step, instead of creating new strings in every loop iteration.
- Less memory overhead, since Python doesn’t have to reallocate space repeatedly.
📌 When to Use .join()
?
✔ If you’re building large strings (e.g., assembling an HTML page, processing large text files).
✔ When formatting logs or reports dynamically.
🚀 Performance Benchmark (Concatenation Test, 100,000 Iterations)
✅ Using +
in a loop: ~1.8 seconds
✅ Using .join()
: ~0.02 seconds (90x faster!)
⚠ When NOT to Use .join()
?
❌ If you’re only concatenating a couple of short strings, +
is fine.
❌ If you’re working with non-string objects, make sure to convert them first:
pythonCopyEditnumbers = [1, 2, 3]
result = " ".join(map(str, numbers)) # Convert to strings before joining
💡 Bottom Line: If you’re building large text data, .join()
is your best friend. Use it everywhere you can for faster, more memory-efficient string handling.
Optimize or Be Left Behind
If your Python code runs slow, don’t panic—just optimize the right way.
✅ Key Takeaways:
- Profile first, optimize second—stop guessing.
- Use built-in functions & NumPy—manual loops are slow.
- Leverage generators to cut memory usage.
- Use multiprocessing to utilize all CPU cores.
💡 Want to flex your Python skills IRL? Check out MadDosh’s Python-themed apparel and wear your coder mindset with pride. 🚀
Staff picks
-
$21.00
Colorful Ape Sunglasses Tee
-
$21.00
Bear Graphic Tee
-
$21.00
Squirrel Sunglasses Tee
-
$21.00
Sloth Tee
-
$21.00
Deer Sunglasses Tee
-
$21.00
Crayon Raccoon Tee
Debugging Circular Imports in Python: Clean Project Layout
Circular imports are one of the most common, annoying, and avoidable problems…
Debugging Python AsyncIO Errors: Event Loop Problems Solved
AsyncIO is deceptively simple—until it isn’t. You’re probably here because you hit…
How to Fix Python Memory Leaks With tracemalloc
Struggling with a Python app that keeps eating up memory? Learn how…
Fixing “ModuleNotFoundError” in Python (Fast Debugging Guide)
Struggling with Python’s dreaded ModuleNotFoundError? This fast debugging guide covers exactly why…
How to Resolve ImportErrors and ModuleNotFoundErrors in Python Projects
Struggling with Python import errors? Learn how to fix ImportError and ModuleNotFoundError…
Stop Writing Python Like JavaScript – Common Mistakes and How to Fix Them
Python and JavaScript are not the same, and yet, I keep seeing…
Leave a Reply