Your cart is currently empty!
Perfect Code Is a Myth. Write Code That Survives
Every software engineer gets bombarded with the idea that their code should be “clean.” Clean code this, clean code that. There’s a cult-like obsession with writing elegant, minimal, beautifully structured code that looks like it was written by a poet rather than an engineer. And honestly? It’s a waste of time.
Instead of obsessing over “clean” code, you should be writing maintainable code. Because the reality is: code doesn’t exist in a vacuum. It lives in messy, evolving, real-world projects where clarity, adaptability, and collaboration matter far more than how aesthetically pleasing your function names are.
Clean Code vs. Maintainable Code: What’s the Difference?
Clean code is mostly about writing aesthetically pleasing, logically structured, and easy-to-read code. That’s nice, but it’s also a personal preference and varies wildly from one developer to another. It prioritizes readability over everything else, sometimes to an extreme degree.
Maintainable code, on the other hand, is practical. It’s about making sure that your code can:
- Be understood by someone who didn’t write it (including future you)
- Be modified without causing a cascade of breakages
- Be scaled without requiring a full rewrite
- Be debugged in minutes, not hours
- Survive long enough to stay useful
The difference? Clean code is idealistic. Maintainable code is pragmatic.
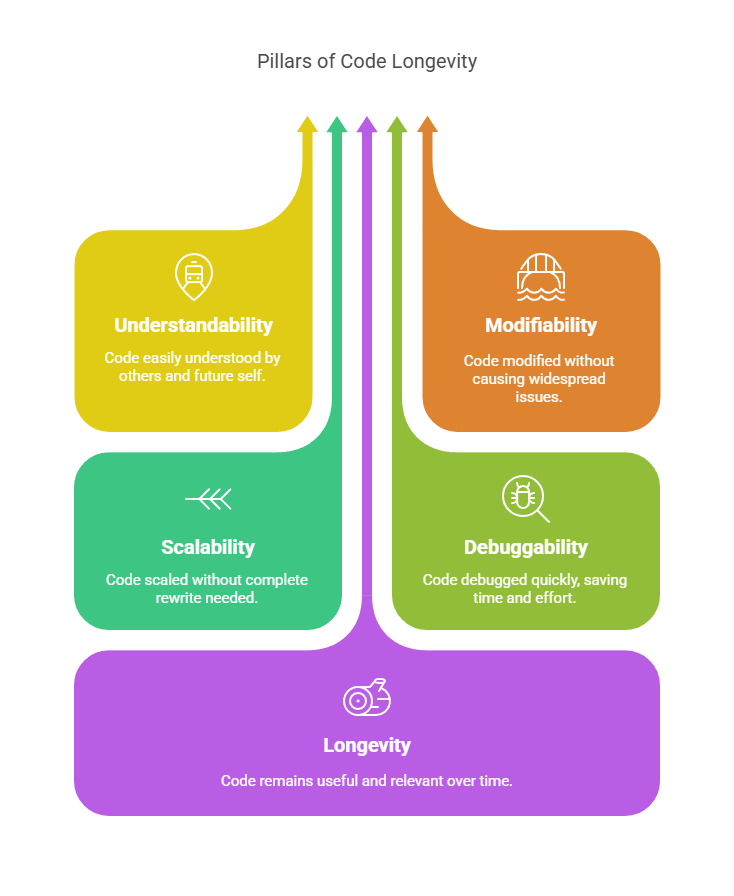
The Problem With “Clean” Code
The biggest issue with clean code is that it’s subjective as hell. What looks clean to you might be confusing to someone else. Ever tried reading someone else’s “clean” code that was so over-abstracted it felt like solving a puzzle? Exactly.
Here’s where clean code goes wrong:
- Over-Engineering — Clean code enthusiasts love refactoring things to the point where a simple function turns into an abstract, over-engineered nightmare. Suddenly, you’re five files deep into a class hierarchy just to find out where a basic string is being formatted.
- Premature Optimization — “Oh, but if we do this now, it’ll be easier in the future!” No. You don’t even know what the future requirements will be. Over-abstracting leads to code that’s harder to change later, not easier.
- Too Many Rules — Some developers treat clean code like it’s a religious doctrine. Every function must be under 10 lines. Every class must follow SOLID to the letter. Every variable name must be an essay. At some point, these rules stop helping and start getting in the way.
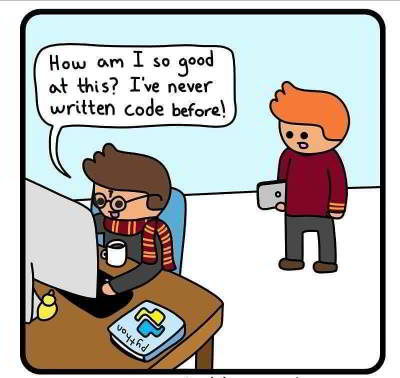
What Maintainable Code Actually Looks Like
Instead of wasting time debating whether a variable name is “clean,” focus on what makes your code maintainable:
1. It’s Obvious, Not Clever
If you have to stop and think about what a piece of code does, it’s already a failure. Clever tricks might make you feel smart, but they make debugging and onboarding a nightmare.
🚫 Bad:
r = [x[::-1] for x in list(map(lambda s: s.strip(), open('file.txt').readlines())) if x]
✅ Good:
with open('file.txt') as f:
lines = f.readlines()
reversed_lines = []
for line in lines:
stripped = line.strip()
if stripped:
reversed_lines.append(stripped[::-1])
It’s not “cleaner” to pack everything into a one-liner. It’s just harder to read and maintain.
2. It’s Boring but Predictable
If you’ve worked on legacy systems, you’ll know that boring, predictable code is a lifesaver. Maintainable code doesn’t have to be exciting — it has to be consistent.
🚫 Bad:
let v = document.querySelectorAll("#items > .thing");
v.forEach(t => doSomething(t));
✅ Good:
const items = document.querySelectorAll("#items > .thing");
items.forEach((item) => doSomething(item));
The second example isn’t revolutionary — it’s just obvious. Maintainable code is written for humans, not just for the compiler.
3. It’s Easy to Change Without Fear
Refactoring should be low-risk. Maintainable code allows you to change one thing without breaking everything else.
🚫 Bad:
public void processUser(String username) {
User u = db.getUser(username);
if (u != null && u.getStatus().equals("active")) {
Notification.send(u.getEmail(), "Welcome back!");
}
}
What if we suddenly need to send an SMS instead of an email? Or check another condition? The logic is too tightly coupled.
✅ Good:
public void processUser(String username) {
User user = db.getUser(username);
if (isActiveUser(user)) {
notifyUser(user);
}
}
private boolean isActiveUser(User user) {
return user != null && user.getStatus().equals("active");
}
private void notifyUser(User user) {
notificationService.send(user.getEmail(), "Welcome back!");
}
Now, if we need to change how we notify users, we only touch one function instead of breaking the entire process.
4. It Documents Itself
Maintainable code doesn’t need a wall of comments — it should explain itself through structure. Comments should be used for why something exists, not what it does.
🚫 Bad:
# Open the file and read the contents
f = open("data.txt", "r")
data = f.read()
f.close()
✅ Good:
with open("data.txt", "r") as file:
data = file.read()
No comment needed, because the code itself is self-explanatory.
Final Thoughts
Writing “clean” code is an overhyped obsession that makes developers focus on perfection instead of practicality. What actually matters is writing maintainable code — code that doesn’t break easily, is easy to read, and can evolve with your project.
Next time you find yourself obsessing over whether your code is “clean” enough, ask yourself: Would someone else be able to modify this without cursing my name? If the answer is yes, congrats — you wrote maintainable code.
Staff picks
-
$21.00
Colorful Ape Sunglasses Tee
-
$21.00
Hedgehog Sunglasses Tee
-
$21.00
Dog Lover Graphic Tee – Labrador Retriever with Sunglasses Unisex T-Shirt
-
$21.00
Frog Sunglasses Tee
-
$21.00
Deer Sunglasses Tee
-
$15.00 – $19.00
Infinite Loop Coffee Mug – Python Developer Gift
How to Resolve ImportErrors and ModuleNotFoundErrors in Python Projects
Struggling with Python import errors? Learn how to fix ImportError and ModuleNotFoundError…
Stop Writing Python Like JavaScript – Common Mistakes and How to Fix Them
Python and JavaScript are not the same, and yet, I keep seeing…
The 2025 Developer Report: 150+ Data Points on Salaries, Skills & Trends
With AI-assisted coding, rising salaries, and shifting work environments, 2025 is shaping…
Top 25 Most Common Python Mistakes (And How to Avoid Them)
Even experienced Python developers fall into common traps—slow loops, bad exception handling,…
Stop Writing Clean Code, Write Maintainable Code Instead
Perfect Code Is a Myth. Write Code That Survives Every software engineer gets…
Why Every Python Developer Should Master List Comprehensions
List comprehensions are one of Python’s most powerful features, making code cleaner,…
Leave a Reply