Your cart is currently empty!
Circular imports are one of the most common, annoying, and avoidable problems in Python development. They crush productivity, break your app, and signal bad architecture.
This guide delivers:
- ✅ Why circular imports happen
- ✅ How to fix them instantly
- ✅ The exact Python project layout that prevents them permanently
By the end, you’ll never Google “circular import fix” again.
Table of Contents
- What Are Circular Imports?
- Why Python Circular Imports Happen
- How to Fix Circular Imports (4 Proven Methods)
- Clean Python Project Layout That Kills Circular Imports
- Best Practices for Dependency Management
- Frequently Asked Questions (FAQs)
- Copy-Paste Project Template (For Immediate Use)
- Circular Imports Are a Code Smell—Fix Them Before They Kill Your Project
- TL;DR
What Are Circular Imports?
Circular imports occur when two or more modules rely on each other to work.
Module A imports something from Module B, and Module B tries to import something back from Module A.
This creates a dependency loop.
Why It Breaks:
- Python executes files top to bottom, loading functions, classes, and variables as it goes.
- If Module B calls back to Module A before it’s finished loading, Python finds partially-built modules.
- You get
ImportError
orAttributeError
because Python can’t find what isn’t ready yet.
Simple Example of Circular Import Hell:
pythonCopyEdit# a.py
from b import func_b
def func_a():
print("Function A")
# b.py
from a import func_a
def func_b():
print("Function B")
What You Get:
pgsqlCopyEditImportError: cannot import name 'func_a' from 'a'
Circular imports are not a bug in Python. They’re a signal that your project’s architecture is broken.
If modules depend on each other like this, you’ve got tight coupling—and that’s the root of the problem.
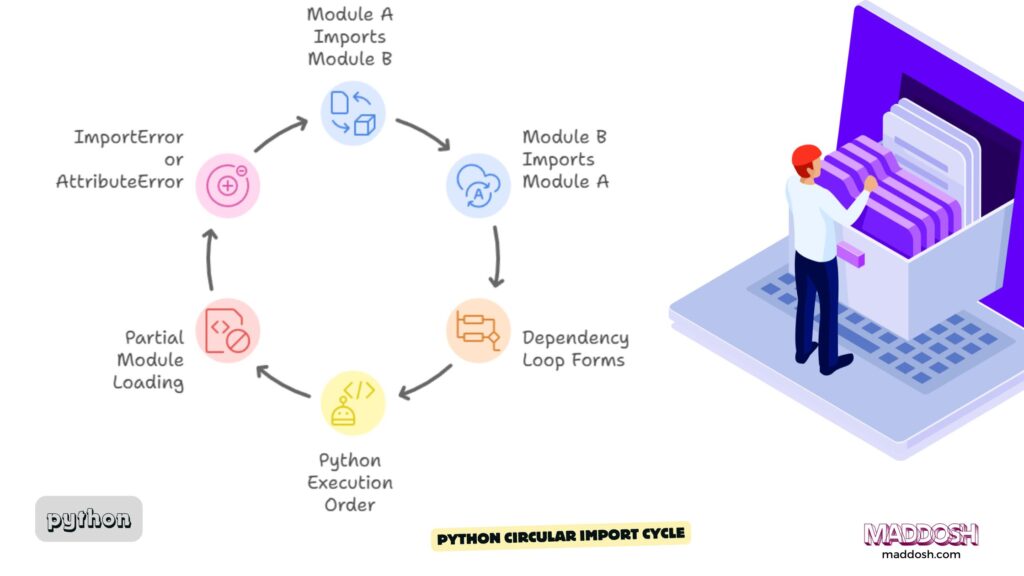
🔥 Quick Example: Broken Imports
pythonCopyEdit# user.py
from post import Post
class User:
pass
# post.py
from user import User
class Post:
pass
What Happens?
shellCopyEditImportError: cannot import name 'User'
Why Python Circular Imports Happen
Python doesn’t load your entire project at once. It:
- Runs a module line-by-line.
- Imports dependencies when it sees the import statement.
- If module A depends on module B, and B depends on A, Python hits an incomplete object. That’s your problem.
If your Python app is throwing weird ImportError
s or AttributeError
s, there’s a good chance you’re stuck in a circular import. Here’s how to confirm it—fast.
1. Read the Stack Trace (Don’t Skip This)
- Look for
ImportError
orAttributeError
. - If the traceback bounces back and forth between the same files, you’ve got a circular import.
- The import loop is usually obvious—read all the way to the bottom.
2. Print the Offending Object
- If an imported function, class, or variable is
None
or missing, that’s your red flag. - It means Python tried to import the module before it finished loading.
Quick Test:
pythonCopyEditfrom some_module import SomeClass
print(SomeClass)
# If it prints None (or raises), you’ve got an incomplete import.
3. Search for Circular Dependencies (Expose the Cycle)
Find two-way imports. They’re the smoking gun.
Use grep
on the command line:
bashCopyEditgrep -rnw . -e 'import '
What to look for:
- Module A imports Module B
- Module B imports Module A
✅ You just found your cycle.
Bonus: Use a Visualizer for Larger Projects
If your project has more than a handful of files, use:
pydeps
for a dependency graphpylint --cyclic-import
to highlight cycles directly
How to Fix Circular Imports (4 Proven Methods)
You fix circular imports by breaking the dependency cycle.
There’s no magic. There’s no hidden Python setting.
You need to rethink how your modules depend on each other and control when and where imports happen.
👉 Here’s the rule:
If two modules need each other, you’re either importing too early or you’ve architected them wrong.
The fixes below aren’t random hacks—they’re repeatable, scalable strategies that work in every Python codebase.
Whether you’re dealing with a simple CLI app or a 10,000-line backend system, these methods stop the import hell.
Method 1: Inline Imports (Move Inside Functions/Methods)
Only import when the function runs, not when the module loads.
Example:
pythonCopyEdit# post.py
def create_post():
from user import User
user = User()
return Post(user)
Why it works: Delays the import until runtime.
Method 2: Refactor Shared Code Into a Third Module
If two modules need each other’s stuff, move it to a third, neutral module.
New Layout:
arduinoCopyEditproject/
├── common.py # shared objects
├── user.py
└── post.py
common.py
pythonCopyEditclass BaseEntity:
pass
Now both user.py
and post.py
import BaseEntity
from common.py
. No cycle.
Method 3: Use Dependency Injection or Interfaces
Don’t tie implementations together. Code against interfaces, not concrete classes.
interfaces.py
pythonCopyEditclass Repository:
def save(self, obj):
pass
user_repository.py
and post_repository.py
both depend on Repository
, but not on each other.
Method 4: importlib (Emergency Use Only)
Import dynamically at runtime.
pythonCopyEditimport importlib
def dynamic_import():
user_module = importlib.import_module('user')
return user_module.User()
Caveat: This is a hack. Refactor instead.
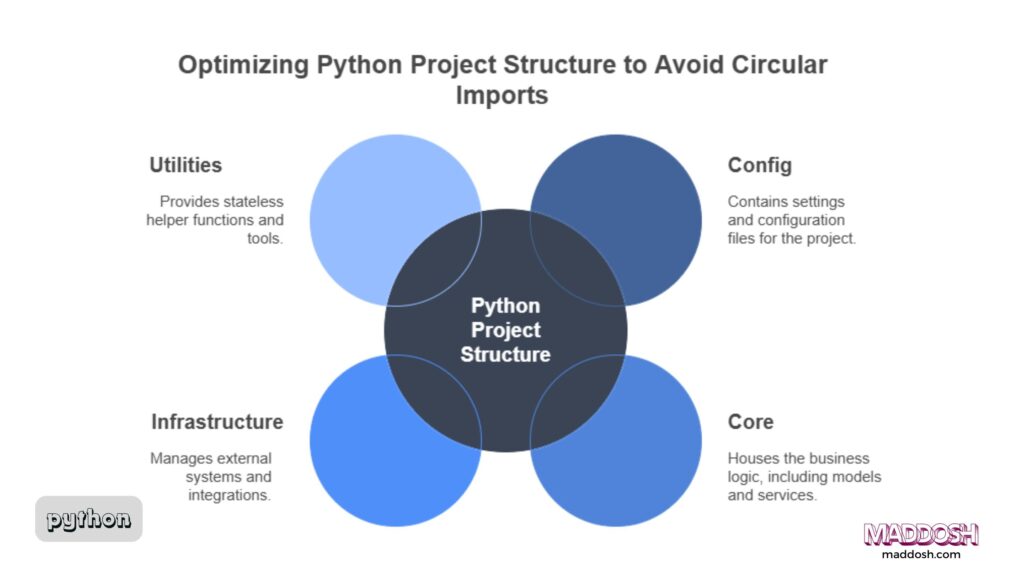
Clean Python Project Layout That Kills Circular Imports
Circular imports = bad project layout. Here’s the clean layout that prevents them.
✅ Recommended Project Structure (2025 Edition)
arduinoCopyEditproject/
├── main.py # entrypoint
├── config/ # settings & config
│ └── config.py
├── core/ # business logic
│ ├── __init__.py
│ ├── models/ # domain objects
│ │ ├── user.py
│ │ └── post.py
│ ├── services/ # service layer
│ │ ├── user_service.py
│ │ └── post_service.py
│ └── validators.py
├── infra/ # external systems
│ ├── db.py
│ └── api_clients.py
└── utils/ # stateless helpers
└── logger.py
✅ Layered Dependencies Flow
- main.py calls core
- core depends on infra and utils
- infra depends on nothing
- utils depend on nothing
Best Practices for Dependency Management
- One-Way Imports Only
- Services should never import models that also import services.
- No Cycles Across Layers
- Domain objects (
models/
) never depend on services.
- Domain objects (
- Flat Utility Modules
utils/
are pure helpers. They never import business logic.
- Use Factories Instead of Direct Imports
- Use factory functions or dependency injection to create instances.
Frequently Asked Questions (FAQs)
❓ Why Does Python Allow Circular Imports?
Because it runs modules dynamically, line by line. You can create cycles, but you shouldn’t.
❓ Does Splitting Code Into More Files Fix Circular Imports?
No. Splitting without clear boundaries just spreads the mess. You need clean dependency rules, not just more files.
❓ What’s the Best Tool to Detect Circular Imports?
pylint --cyclic-import
pydeps
(to visualize dependencies)snakefood
(if you like old-school graphing)
Copy-Paste Project Template (For Immediate Use)
markdownCopyEditproject/
├── main.py
├── config/
│ └── config.py
├── core/
│ ├── models/
│ │ └── __init__.py
│ ├── services/
│ │ └── __init__.py
├── infra/
│ └── db.py
└── utils/
└── logger.py
Example main.py
pythonCopyEditfrom core.services.user_service import UserService
def main():
user_service = UserService()
user_service.create_user("Jane Doe")
if __name__ == "__main__":
main()
Circular Imports Are a Code Smell—Fix Them Before They Kill Your Project
Circular imports are not an edge case. They’re a symptom that your codebase is poorly structured. Ignore them, and they’ll blow up in production—usually when you can’t afford the downtime.
But here’s the thing:
🛠️ Circular imports are 100% preventable.
You don’t need hacks. You need clean architecture, clear separation of concerns, and a disciplined project layout.
Here’s Your Action Plan:
- Fix the current circular imports using inline imports or by refactoring shared logic.
- Audit your project dependencies—if modules are too tightly coupled, untangle them now.
- Restructure your Python project using the layered layout in this guide.
- Enforce one-way dependency rules—no backflow. Ever.
- Document your architecture so new devs don’t reintroduce the same mistakes.
If you can do that, circular imports won’t just be solved. They’ll be impossible.
TL;DR
- Circular imports = broken architecture
- Fix them by refactoring, not patching
- Use a layered project structure to avoid cycles
Staff picks
-
$21.00
Squirrel Sunglasses Tee
-
$21.00
Crayon Raccoon Tee
-
$21.00
Zebra Sunglasses Tee
-
$21.00
Colorful Fox Tee
-
$21.00
Dog Lover Graphic Tee – Labrador Retriever with Sunglasses Unisex T-Shirt
-
$21.00
Deer Sunglasses Tee
Debugging Circular Imports in Python: Clean Project Layout
Circular imports are one of the most common, annoying, and avoidable problems…
Debugging Python AsyncIO Errors: Event Loop Problems Solved
AsyncIO is deceptively simple—until it isn’t. You’re probably here because you hit…
How to Fix Python Memory Leaks With tracemalloc
Struggling with a Python app that keeps eating up memory? Learn how…
Fixing “ModuleNotFoundError” in Python (Fast Debugging Guide)
Struggling with Python’s dreaded ModuleNotFoundError? This fast debugging guide covers exactly why…
How to Resolve ImportErrors and ModuleNotFoundErrors in Python Projects
Struggling with Python import errors? Learn how to fix ImportError and ModuleNotFoundError…
Stop Writing Python Like JavaScript – Common Mistakes and How to Fix Them
Python and JavaScript are not the same, and yet, I keep seeing…
Leave a Reply