Your cart is currently empty!
In Python, the import
statement is fundamental for modular programming, allowing developers to organize code into reusable modules and packages. This mechanism enables the inclusion of external libraries and internal code modules, promoting code reusability and maintainability.
However, during the import process, developers may encounter two common exceptions: ImportError
and ModuleNotFoundError
. Understanding these exceptions is crucial for effective debugging and ensuring the smooth execution of Python applications.
ImportError
: This exception is raised when an import statement fails to load a module. This can occur due to various reasons, such as syntax errors within the module, missing dependencies, or issues with the module’s initialization.
ModuleNotFoundError
: Introduced in Python 3.6 as a subclass of ImportError
, this specific exception is raised when the Python interpreter is unable to locate the module specified in the import statement. Common causes include the module not being installed, incorrect module names, or misconfigured module search paths.
In this article, we will delve into the causes of these exceptions and provide practical solutions to resolve them, ensuring robust and error-free Python code.
Table of Contents
- Understanding ImportError and ModuleNotFoundError in Python
- Debugging Common Causes of Import Errors in Python
- 1. Module Not Installed
- 2. Incorrect Python Environment
- 3. Typographical Errors in Import Statements
- 4. Naming Conflicts with Standard Libraries
- 5. Relative vs. Absolute Imports
- Diagnosing Import Errors in Python
- 1. Checking Installed Modules
- 2. Verifying Python Environment
- 3. Inspecting sys.path
- 4. Identifying Naming Conflicts
- 1. Module Not Installed
- Final Thoughts
Understanding ImportError and ModuleNotFoundError in Python
Python’s import system is fundamental for modular programming, enabling code reuse and organization. However, import errors like ImportError
and ModuleNotFoundError
can disrupt development. Understanding these errors and their root causes is essential for efficient debugging and smoother workflows.
What Is an ImportError in Python?
An ImportError
occurs when Python attempts to import a module but encounters an issue that prevents it from loading. Unlike ModuleNotFoundError
, this doesn’t necessarily mean the module is missing—it could be related to dependencies, syntax issues, or circular imports.
4 Common Causes of ImportError:
- Syntax errors in the module – If the imported module contains syntax errors, Python will fail to load it. Use
python -m py_compile <module>.py
to check for syntax errors. - Missing dependencies – If a module depends on another package that isn’t installed, it will fail to load. Use
pipdeptree
to check for missing dependencies. - Circular imports – If two modules import each other recursively, Python might throw an
ImportError
. Refactor the code to avoid cyclic dependencies by moving imports inside functions where necessary. - C extension issues – Some Python modules have compiled components (e.g.,
.so
or.pyd
files). If dependencies for these compiled extensions are missing, the module won’t load. Useldd <compiled_module>.so
(Linux/macOS) ordumpbin /DEPENDENTS <compiled_module>.pyd
(Windows) to check for missing dependencies.
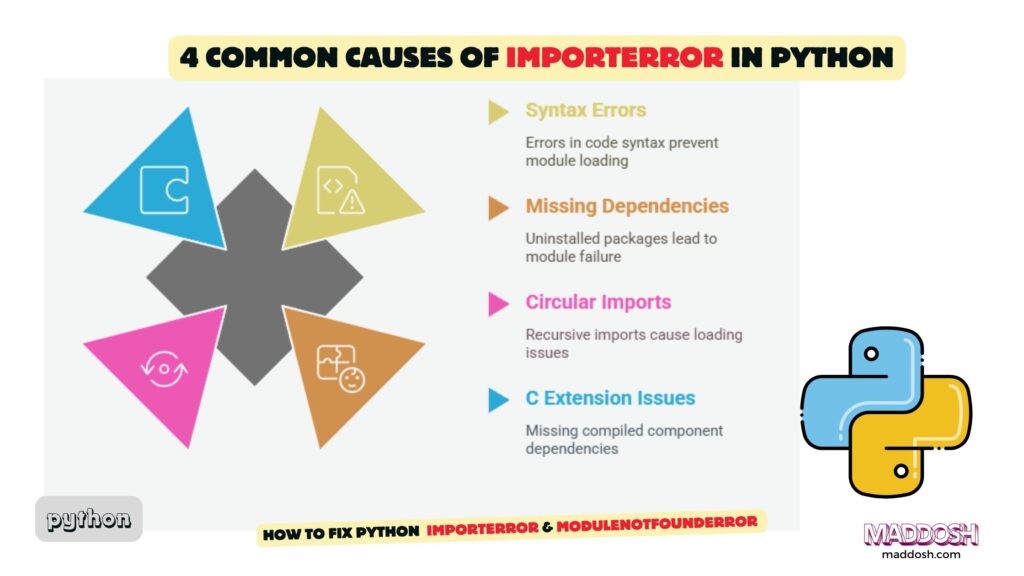
What Is a ModuleNotFoundError in Python?
A ModuleNotFoundError
is a subclass of ImportError
introduced in Python 3.6. It specifically occurs when Python cannot locate the specified module in its import paths.
How ModuleNotFoundError Differs from ImportError:
ModuleNotFoundError
occurs when the module does not exist in the Python environment or is not found insys.path
.ImportError
occurs when the module is found but cannot be loaded due to internal issues (syntax errors, dependencies, etc.).
4 Common Causes of ModuleNotFoundError:
- The module isn’t installed – Verify installation with
pip show <module-name>
orpip list | grep <module-name>
. - Incorrect Python environment – If multiple Python environments exist, the module may be installed in a different one. Use
which python
(Linux/macOS) orwhere python
(Windows) to confirm the active environment. - Incorrect import statement – Ensure the import statement matches the module name exactly (Python is case-sensitive).
- Module isn’t in Python’s search path (
sys.path
) – Check withimport sys; print(sys.path)
and update paths if needed.
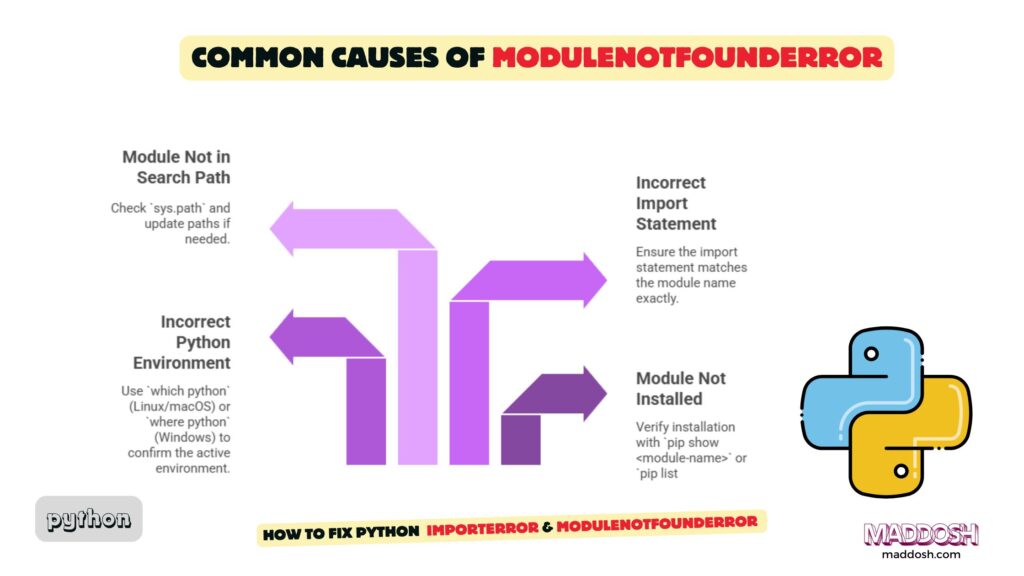
Debugging Common Causes of Import Errors in Python
1. Module Not Installed
A missing module is one of the most common causes of import errors.
How to Check if a Module is Installed:
pip list | grep <module-name>
pip show <module-name>
How to Fix:
pip install <module-name>
python3 -m pip install <module-name>
2. Incorrect Python Environment
If a module is installed but Python still can’t find it, you might be using the wrong Python environment.
How to Check:
which python # macOS/Linux
where python # Windows
pip -V # Check pip version
How to Fix:
source venv/bin/activate # macOS/Linux
venv\Scripts\activate # Windows
python3 -m pip install <module-name>
3. Typographical Errors in Import Statements
Python’s import system is case-sensitive, so even small typos can cause errors.
Common Mistakes:
import json
✅ vs.import Json
❌import numpy
✅ vs.import numppy
❌import config_parser
❌ (should beconfigparser
)
How to Fix:
- Double-check spelling.
- Use an IDE with autocomplete.
- Run
pip list | grep <partial-module-name>
to verify spelling.
4. Naming Conflicts with Standard Libraries
If you name a script random.py
, it can conflict with Python’s built-in random
module.
How to Check:
import random
print(random.__file__)
How to Fix:
- Rename your file.
- Delete
__pycache__
directories.
5. Relative vs. Absolute Imports
Using relative imports incorrectly can break your code.
Best Practices:
- Use absolute imports:
from mypackage.mymodule import my_function
- Avoid relative imports unless necessary:
from .mymodule import my_function # Can cause issues
Diagnosing Import Errors in Python
When encountering ImportError
or ModuleNotFoundError
, the first step is to diagnose the root cause before applying a fix. Python provides several tools and commands to help identify issues with imports. Below are detailed steps for diagnosing and troubleshooting common import errors.
1. Checking Installed Modules
Before assuming something is broken, verify whether the module is installed in the current Python environment. Sometimes, a package might be missing or installed in a different environment.
How to Check Installed Modules:
- List all installed packages: bashCopyEdit
pip list
This will show all packages installed in the current Python environment. - Check details of a specific module: bashCopyEdit
pip show <module-name>
This provides additional information, including the installed version and its location. - Search for a specific module: bashCopyEdit
pip list | grep <module-name> # macOS/Linux pip list | findstr <module-name> # Windows
This helps confirm whether the module exists in the current environment.
How to Fix:
If the module is not listed, install it using:
bashCopyEditpip install <module-name>
For Python 3:
bashCopyEditpython3 -m pip install <module-name>
If the package is installed but still not found, you might be using the wrong Python environment. Move on to Step 2.
2. Verifying Python Environment
Many import errors occur because the module is installed in a different Python environment than the one currently active. This is especially common when using virtual environments or multiple Python versions.
How to Check Which Python Version is Active:
- Check Python version: bashCopyEdit
python --version python3 --version # If using Python 3 explicitly
Ensure that you are using the correct Python version where the package is installed. - Check Python interpreter path: bashCopyEdit
which python # macOS/Linux where python # Windows
This displays the path of the Python binary in use. - Check the active virtual environment: bashCopyEdit
echo $VIRTUAL_ENV # macOS/Linux echo %VIRTUAL_ENV% # Windows
If this command returns nothing, you are likely using the system-wide Python environment instead of a virtual environment.
How to Fix:
- If the module is installed in a different environment, activate the correct environment before running Python: bashCopyEdit
source venv/bin/activate # macOS/Linux venv\Scripts\activate # Windows
- If the module is installed globally but your script runs in a virtual environment, install it inside the correct virtual environment: bashCopyEdit
python -m pip install <module-name>
- If you are using
conda
, ensure you are in the correct conda environment: bashCopyEditconda activate <your-env> conda list | grep <module-name>
3. Inspecting sys.path
If a module isn’t found, it might not be in Python’s search path. Python uses sys.path
to determine where to look for installed modules.
How to Check Python’s Module Search Paths:
pythonCopyEditimport sys
print(sys.path)
This command prints a list of directories where Python looks for modules.
What to Look For:
- If the directory where your module is installed is missing from
sys.path
, Python will not be able to locate it. - If a different Python version’s
site-packages
directory appears, you are likely running the wrong interpreter.
How to Fix:
If the module’s directory is missing from sys.path
, you can manually add it:
pythonCopyEditimport sys
sys.path.append("/path/to/your/module")
However, modifying sys.path
dynamically should only be a temporary fix. A better approach is to correctly install the package or adjust the Python environment.
If your module is inside a package, ensure the parent directory contains an __init__.py
file. Without it, Python may not recognize the directory as a package.
4. Identifying Naming Conflicts
Python might not be importing the correct module due to naming conflicts. If your script or directory shares the same name as a standard library module, Python might accidentally import your local file instead of the built-in module.
How to Check if There’s a Naming Conflict:
pythonCopyEditimport module_name
print(module_name.__file__)
This command prints the file path of the imported module.
What to Look For:
- If the path points to a file inside your project directory rather than the standard library or
site-packages
, you have a naming conflict.
How to Fix:
- Rename your script or module if it has the same name as a standard library module (e.g.,
random.py
,json.py
,email.py
). - Delete cached files that may be interfering with imports: bashCopyEdit
rm -rf __pycache__ # macOS/Linux del /s /q __pycache__ # Windows
- Ensure imports are correctly referencing the intended module by using absolute imports instead of relative ones.
Summary
When troubleshooting import errors, follow these steps:
✅ Check if the module is installed with pip list
or pip show <module-name>
.
✅ Verify the Python environment to ensure you’re running the correct interpreter.
✅ Inspect sys.path
to confirm Python is searching the correct directories.
✅ Look for naming conflicts to avoid accidentally shadowing built-in modules.
By systematically diagnosing and resolving these issues, you can avoid import errors and keep your Python code running smoothly. 🚀
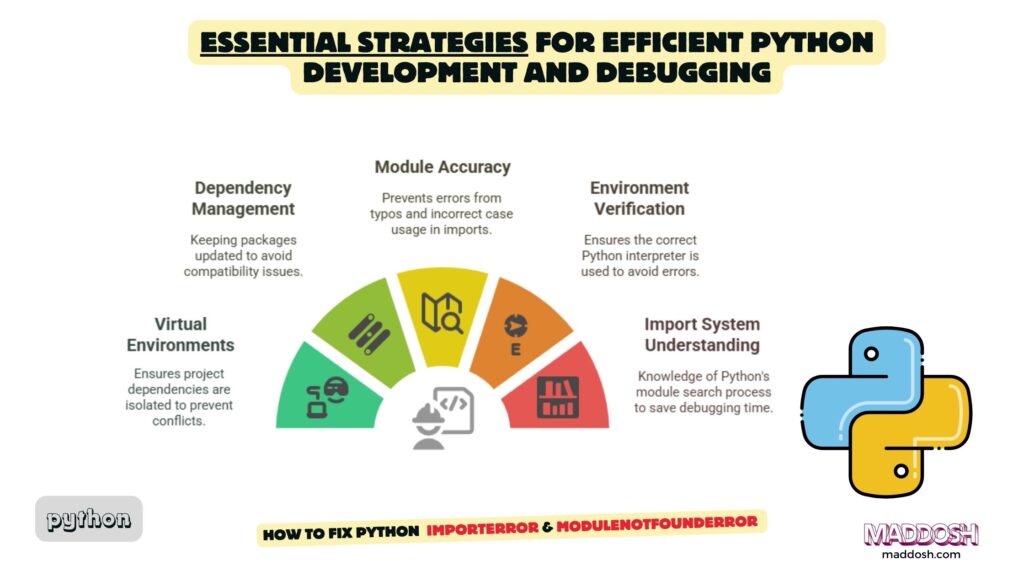
Final Thoughts
Python import errors can be frustrating, especially when they disrupt your workflow. However, most of these issues are preventable with a structured approach to managing your project’s dependencies and environment.
Here’s a quick recap of key takeaways:
- Always use virtual environments – This ensures your project dependencies are isolated and prevents conflicts with system-wide installations.
- Keep dependencies up to date – Regularly running
pip list --outdated
and updating packages prevents compatibility issues. - Double-check module names and paths – Typos and incorrect case usage in import statements are common pitfalls.
- Verify your Python environment – Running the wrong Python interpreter is one of the most frequent causes of
ModuleNotFoundError
. Always check withwhich python
(Linux/macOS) orwhere python
(Windows). - Understand Python’s import system – Knowing how Python searches for modules via
sys.path
and how to modify it when necessary can save hours of debugging.
By adopting these best practices, you can significantly reduce the chances of encountering ImportError
and ModuleNotFoundError
, keeping your Python projects running smoothly.
Need Some Python-Inspired Gear?
If you’re constantly troubleshooting imports and debugging code, why not represent your passion for Python with some developer merch? Check out our Python-themed hoodies, t-shirts, and accessories
👉 Shop Now
Staff picks
-
$21.00
Koala Sunglasses Tee
-
$21.00
Tech Cat Unisex Tee
-
$21.00
Sloth Tee
-
$21.00
Frog Sunglasses Tee
-
$21.00
Colorful Ape Sunglasses Tee
-
$21.00
Wolf Tee – Colorful Crayon Drawing, Unisex Heavy Cotton Shirt
Debugging Circular Imports in Python: Clean Project Layout
Circular imports are one of the most common, annoying, and avoidable problems…
Debugging Python AsyncIO Errors: Event Loop Problems Solved
AsyncIO is deceptively simple—until it isn’t. You’re probably here because you hit…
How to Fix Python Memory Leaks With tracemalloc
Struggling with a Python app that keeps eating up memory? Learn how…
Fixing “ModuleNotFoundError” in Python (Fast Debugging Guide)
Struggling with Python’s dreaded ModuleNotFoundError? This fast debugging guide covers exactly why…
How to Resolve ImportErrors and ModuleNotFoundErrors in Python Projects
Struggling with Python import errors? Learn how to fix ImportError and ModuleNotFoundError…
Stop Writing Python Like JavaScript – Common Mistakes and How to Fix Them
Python and JavaScript are not the same, and yet, I keep seeing…
Leave a Reply